[백준 2667] 단지 번호 붙이기 (실버 1) 문제 풀이- 자바 Java
2023. 1. 7. 12:15ㆍ네카라쿠배 취준반 - 프로그래머스 문제 풀이
0. 자세한 설명은 YouTube 영상으로
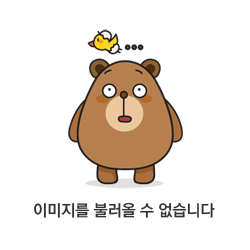
1. visited 배열을 사용한 정석 풀이
// ver1
import java.util.*;
import java.io.*;
class Main {
final static int MAX = 25 + 10;
static boolean[][] graph;
static boolean[][] visited;
static int countPerDanji;
static int dirY[] = { 1, -1, 0, 0 };
static int dirX[] = { 0, 0, 1, -1 };
static void dfs(int y, int x) {
visited[y][x] = true;
countPerDanji++;
for (int i = 0; i < 4; i++) {
int newY = y + dirY[i];
int newX = x + dirX[i];
if (!visited[newY][newX] && graph[newY][newX])
dfs(newY, newX);
}
}
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int N = Integer.parseInt(br.readLine());
graph = new boolean[MAX][MAX];
visited = new boolean[MAX][MAX];
for (int i = 1; i <= N; i++) {
String s = br.readLine();
for (int j = 1; j <= N; j++)
graph[i][j] = s.charAt(j - 1) == '1';
}
ArrayList<Integer> countList = new ArrayList<>();
for (int i = 1; i <= N; i++)
for (int j = 1; j <= N; j++)
if (graph[i][j] && !visited[i][j]) {
countPerDanji = 0;
dfs(i, j);
countList.add(countPerDanji);
}
System.out.println(countList.size());
Collections.sort(countList);
for (int i = 0; i < countList.size(); i++)
System.out.println(countList.get(i));
br.close();
}
}
2. graph 배열만 사용한 풀이
// ver 2
import java.util.*;
import java.io.*;
class Main {
final static int MAX = 25 + 10;
static boolean[][] graph;
static int countPerDanji;
static int dirY[] = { 1, -1, 0, 0 };
static int dirX[] = { 0, 0, 1, -1 };
static void dfs(int y, int x) {
graph[y][x] = false;
countPerDanji++;
for (int i = 0; i < 4; i++) {
int newY = y + dirY[i];
int newX = x + dirX[i];
if (graph[newY][newX])
dfs(newY, newX);
}
}
public static void main(String[] args) throws IOException {
// 0. 입력 및 초기화
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int N = Integer.parseInt(br.readLine());
graph = new boolean[MAX][MAX];
// 1. 그래프 정보 입력
for (int i = 1; i <= N; i++) {
String s = br.readLine();
for (int j = 1; j <= N; j++)
graph[i][j] = s.charAt(j - 1) == '1';
}
// 2. (1, 1) 부터 (N, N)까지 dfs 수행
ArrayList<Integer> countList = new ArrayList<>();
for (int i = 1; i <= N; i++)
for (int j = 1; j <= N; j++)
if (graph[i][j]) {
countPerDanji = 0;
dfs(i, j);
countList.add(countPerDanji);
}
// 3. 출력
System.out.println(countList.size());
Collections.sort(countList);
for (int i = 0; i < countList.size(); i++)
System.out.println(countList.get(i));
br.close();
}
}
'네카라쿠배 취준반 - 프로그래머스 문제 풀이' 카테고리의 다른 글
[백준 1764] 듣보잡 (실버 4) 문제 풀이- 자바 Java 해시 (0) | 2023.01.14 |
---|---|
[백준 10816] 숫자 카드 2(실버 4) 문제 풀이- 파이썬 python (0) | 2023.01.12 |
백준 DFS 입문 문제 추천 (나 빼고 다 풀었다는 DFS 문제 모음) (7) | 2023.01.07 |
[백준 1012] 유기농 배추 (실버 3) 문제 풀이- 파이썬 python (2) | 2023.01.05 |
[백준 2606] 바이러스 (실버 3) 문제 풀이- 자바 Java (0) | 2022.12.31 |